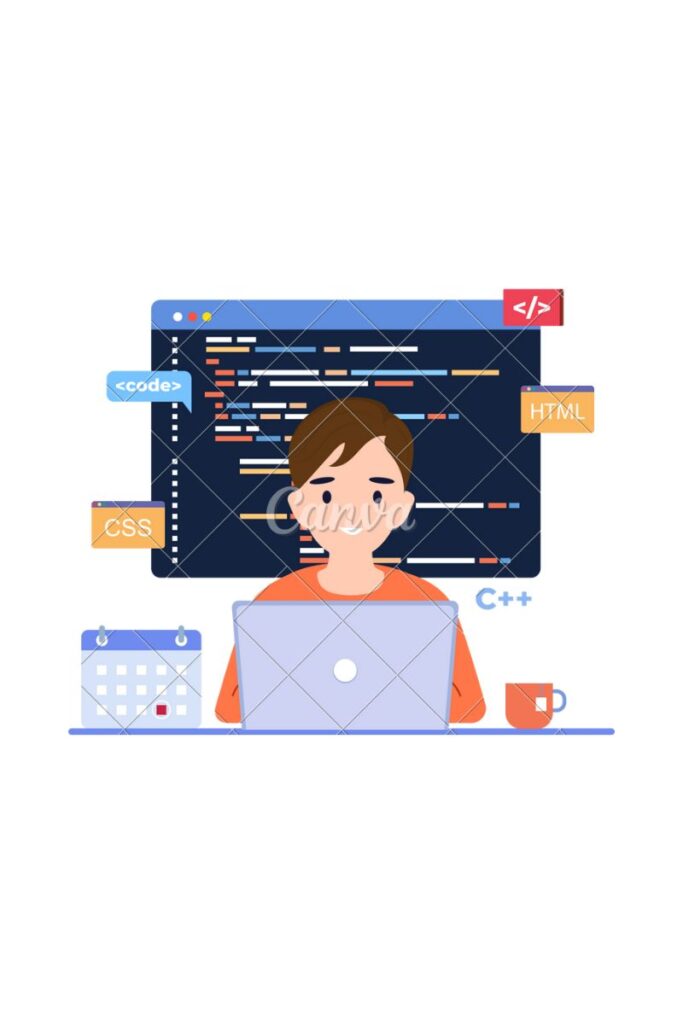
JavaScript is the most widely used front-end language. Although new frameworks keep emerging, none have been able to replace JavaScript, as all frameworks are built upon it.
To become a successful front-end developer, having a strong understanding of JavaScript is essential.
In this blog, we will take on the 100-day JavaScript challenge. Below is the roadmap for the journey!”**
This version improves clarity, grammar, and flow while maintaining a professional yet engaging tone. Let me know if you want further refinements! 🚀
Week 1-2: JavaScript Basics & Inbuilt Methods
Week 1: Getting Comfortable with JavaScript
Day 1: Introduction & Setup
- What is JavaScript? Why is it important?
- Setting up VS Code & Browser DevTools
- Writing first JavaScript program
Day 2: Variables & Data Types - var, let, const
- Primitive vs. Reference types
- Type conversion (Number(), String(), Boolean())
Day 3: Operators & Expressions - Arithmetic, Logical, Comparison Operators
- typeof, instanceof, isNaN()
- Implicit vs. Explicit type conversion
Day 4: Strings & Basic String Methods - length, charAt(), indexOf(), toUpperCase(), toLowerCase()
- Template literals
Day 5: Advanced String Methods - slice(), substring(), replace(), split(), trim(), includes()
Day 6: Numbers & Math Methods - parseInt(), parseFloat(), toFixed()
- Math.round(), Math.ceil(), Math.floor(), Math.random()
Day 7: Review & Hands-on Practice
Week 2: Arrays & Objects
Day 8-10: Arrays & Methods (push(), pop(), map(), filter(), reduce())
Day 11-12: Objects & Methods (Object.keys(), Object.values(), Object.entries())
Day 13: Object vs. Array Use Cases
Day 14: Mini Project – To-Do List
Week 3-4: Functions, Loops & DOM
Day 15-19: Functions, Scope, Closures, Higher-Order Functions
Day 20-24: DOM Manipulation, Events, Handling User Input
Day 25-28: Local Storage, Error Handling, Mini Project – Shopping List
Week 5-6: Asynchronous JS & Advanced Topics
Day 29-35: Callbacks, Promises, Async/Await, Fetch API, Timers
Day 36-42: Prototypes, ES6+ Classes, Modules, Event Loop, Mini Project
Week 7-12: Deep Dives & Real-World Projects
- Design Patterns, Performance Optimizations
- WebSockets, Security Best Practices
- Testing with Jest
- Final Project: Full-Stack Dashboard
Leave a Reply